Java Programming For Beginners: Step By Step Lesson #4 || CREATING A SIMPLE CALCULATOR PROGRAM USING ECLIPSE IDE (By @kaffy22)
What Will I Learn?
- You will learn how to create a simple arithmetic calculator Java program.
- You will learn how to use Scanner to input data from keyboard while running a program.
Requirements
- Computer system
- JDK must be installed
- Eclipse IDE
Difficulty
- The difficulty is just like our previous lessons. So its Basic
Tutorial Contents
Welcome back, budding programmers. We had a great class in our previous lesson. And I believe you're finally agreeing with me that programming is easy and that that it's not gonna be hard for you to become a great programmer.
Today's class is much like our previous one. We are going to create a simple calculator that can add two integer numbers supplied by the user. This time, we are not going to assign values to our variables. The user will input his preferred values while running the program. Now let's get to it guys!
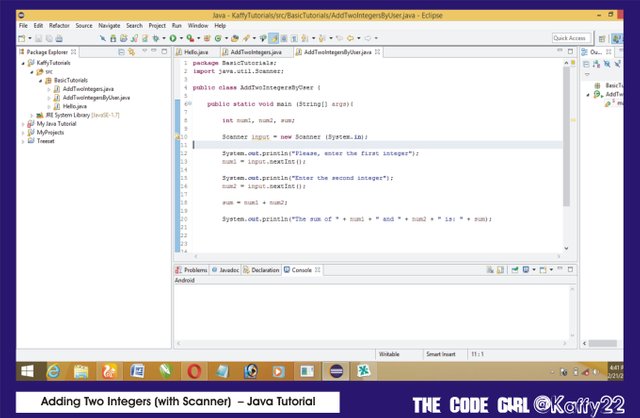
Our program code on Eclipse IDE
Let's take a look at the code vividly.
The Code
package BasicTutorials;
import java.util.Scanner;
public class AddTwoIntegersByUser {
public static void main (String[] args){
int num1, num2, sum;
Scanner input = new Scanner (System.in);
System.out.println("Please, enter the first integer");
num1 = input.nextInt();
System.out.println("Enter the second integer");
num2 = input.nextInt();
sum = num1 + num2;
System.out.println("The sum of " + num1 + " and " + num2 + " is: " + sum);
}
}
You see how simple that lines of code look? Like I said, this program looks much like the program from our previous lesson. It's time I explain each line of code and how it works.
package BasicTutorials;
This is just a package name. Its where our basic tutorials are saved. I already explained this before.
import java.util.Scanner;
This import statement which introduces the Scanner class from the util package to the program. This Scanner class is what we'll be using to input data from keyboard and in printing our output to the output window.
public class AddTwoIntegersByUser {
This is the program class. The AddTwoIntegersByUser is the program class and that represents the name of the program as stored when creating the class.
public static void main(String[] args) {
This is the program main method.
int num1, num2, sum;
This line introduces our variables to the program. We've just declared our variables num1, int num2 and sum with datatype int (integer) as needed in the program. I've explained different methods of declaring variables in Java programming in the previous lesson.
Scanner input = new Scanner (System.in);
Declaring reference variable of type Scanner. This helps helps us in inputting data from keyboard while running the program.
System.out.println("Please, enter the first integer");
A print statement asking the user to input the first integer through the keyboard.
num1 = input.nextInt();
This line stores whatever the user input from the keyboard as the first variable, num1
System.out.println("Enter the second integer");
A print statement asking the user to input the second integer through the keyboard.
num2 = input.nextInt();
This line stores whatever the user input from the keyboard as the second variable, num2
sum = num1 + num2;
Arithmetic operation takes place here and the result is stored as sum
System.out.println("The sum of " + num1 + " and " + num2 + " is: " + sum);
A print statement that prepares our result on the output window.
That's it. We've just created a simple calculator that adds two integers as supplied by the user. Running this program, we must have these outputs below.
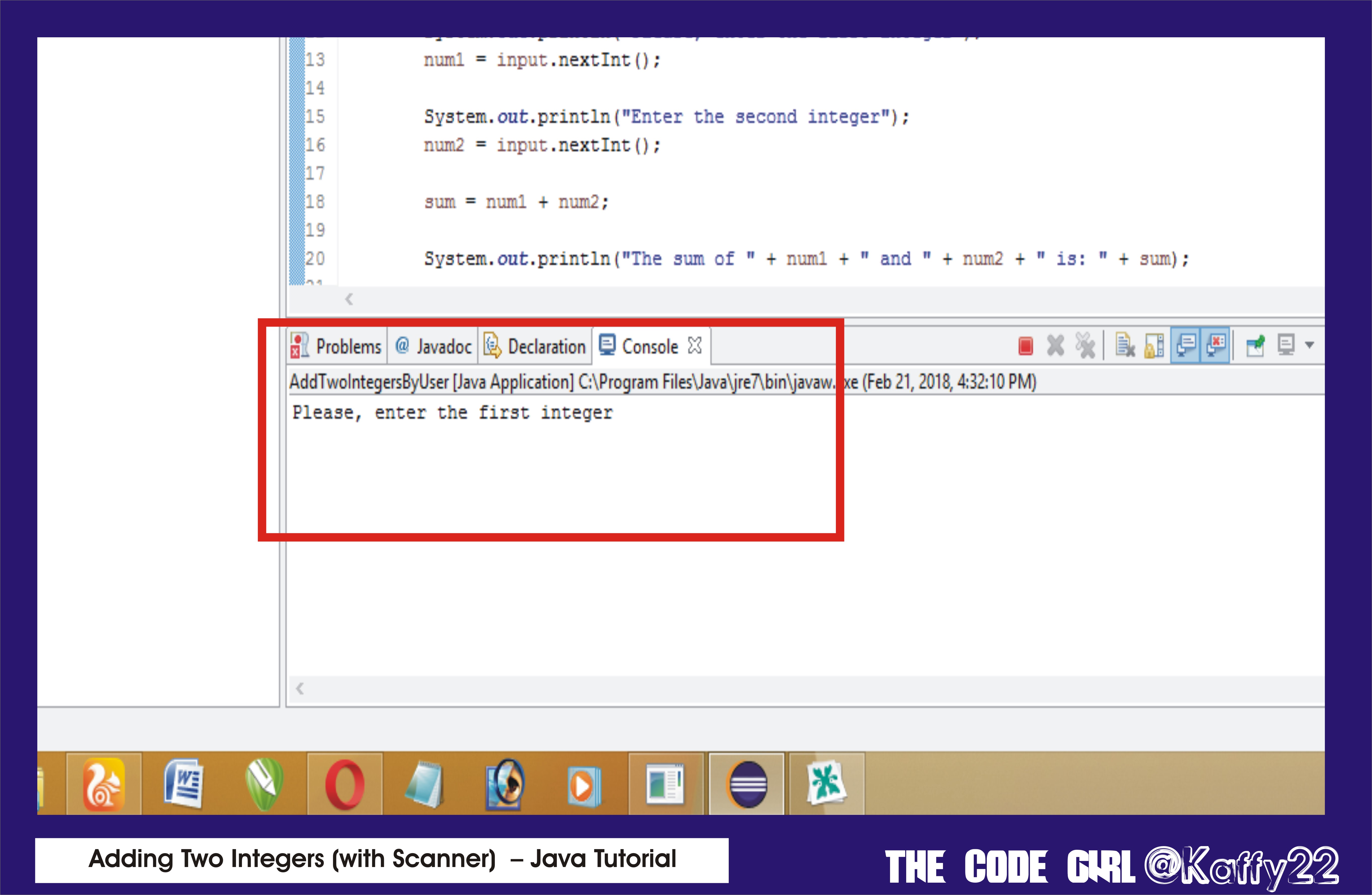
Asking the user to supply the first integer
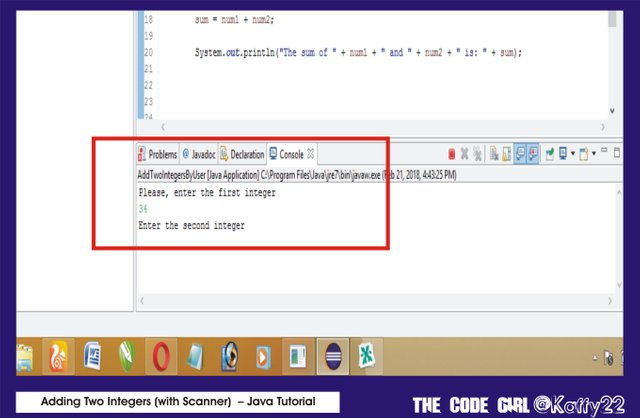
Asking the user to supply the second integer
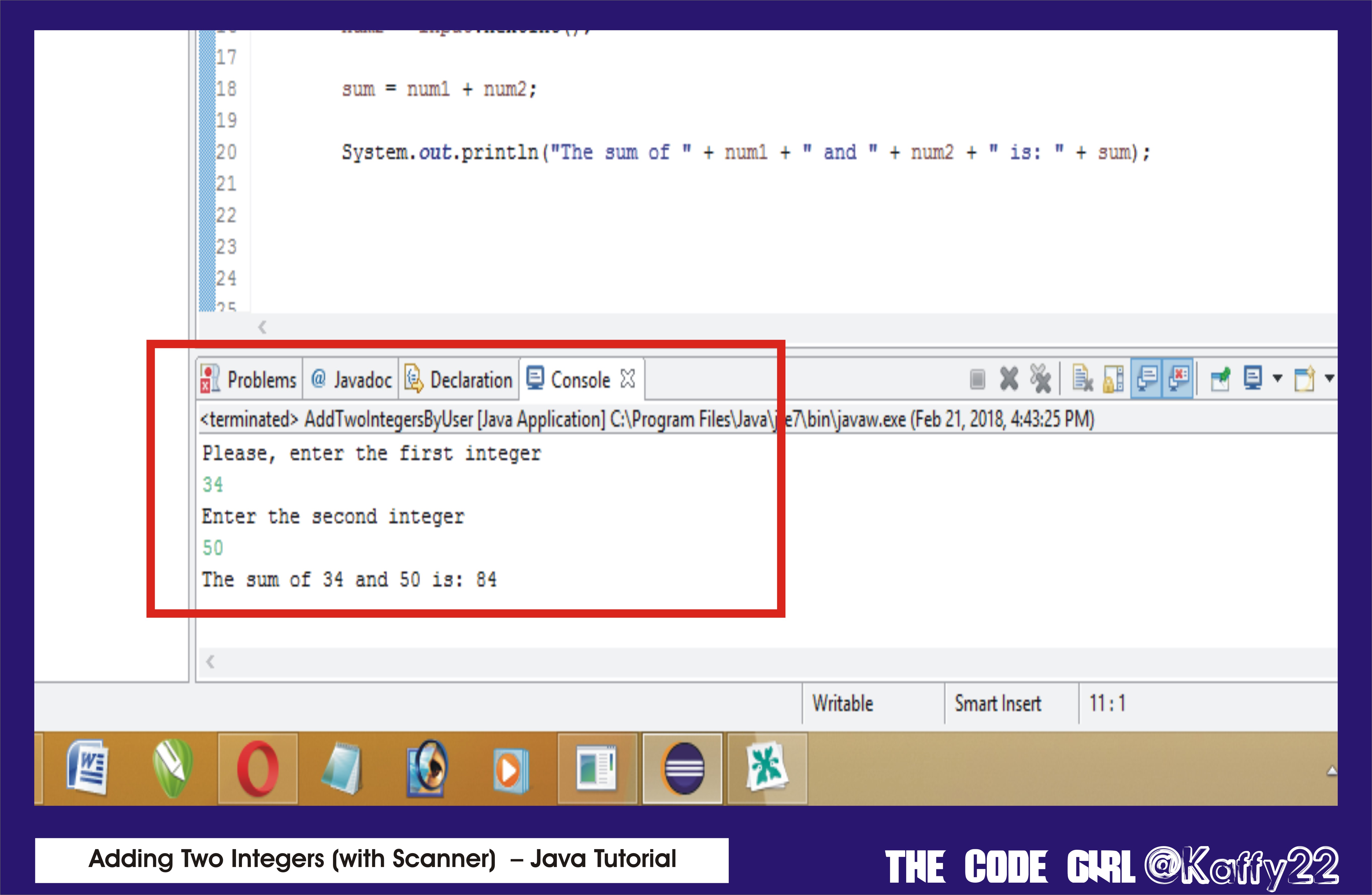
The result is then shown on the output console
This is where we are going to stop for now, we'll continue in the next class. FOLLOW THIS BLOG FOR SUBSEQUENT LESSONS.
Like I do tell you guys, programming is not hard, programming is not easy, your approach determines which of its side programming shows to you - easy or hard
Be sure to leave a comment and Upvote. A resteem is also appreciated.
Curriculum
- Java Programming For Beginners: Step By Step Lesson #3 || CREATING A SIMPLE ARITHMETIC PROGRAM USING ECLIPSE IDE
- Java Programming For Beginners: Step By Step Lesson #2 || Creating Our First Project And Package
- Java Programming For Beginners: Step By Step Lesson #1 || Setting Up Your JDK & Eclipse IDE
Congratulations @kaffy22! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
To support your work, I also upvoted your post!
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Congratulations @kaffy22! You received a personal award!
Click here to view your Board
Congratulations @kaffy22! You received a personal award!
You can view your badges on your Steem Board and compare to others on the Steem Ranking
Vote for @Steemitboard as a witness to get one more award and increased upvotes!